During a strange period at work, I found myself contributing to three different JavaScript based web applications over less than 6 months. All three applications used different “frameworks” (realizing that one of these is not considered a framework) – Angular, React, and Vue. In an effort to keep my web application development skills fresh, I have been building small applications, one of which I made using React and would like to transition into a Docker container.
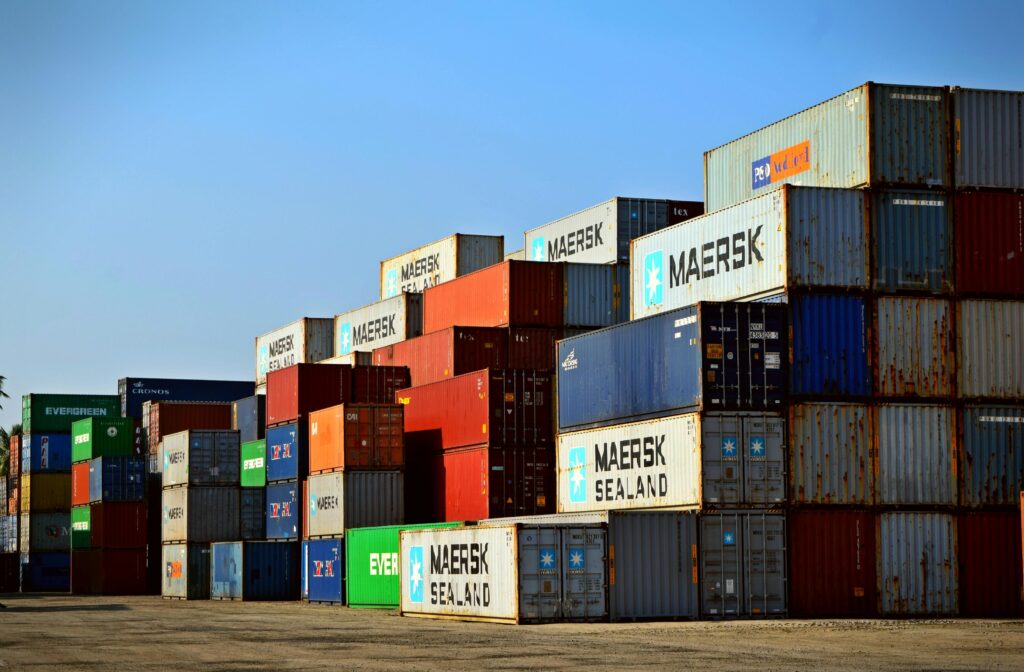
This guide starts with an existing React app, albeit a very basic app with very few elements, and creates a Docker image for that app.
What files to add to your React project to create a Docker image
- Open the folder containing all the files of your React app and create a new file called Dockerfile
- We’re going to include 5 lines of code in our Dockerfile to create a Docker image and run our React app within a container
FROM node:20.10 | The FROM line defines the parent image, sort of like the foundation of your new image. Use Docker Hub website to find the base images to use for your project. For a React app we need Node. The syntax is <image>:<tag> where the tag is the version of the image. |
WORKDIR /example-app/ | The WORKDIR line defines the working directory for any following commands. |
COPY public /public COPY src /src COPY package.json / | Each COPY command moves a piece of the React app, including the package.json file into the working directory |
RUN npm install | The RUN instruction executes package.json using npm install to create all dependencies |
CMD [“npm”, “start”] | The CMD instruction defines the command to be executed when starting the container based on the image. |
How to build the Docker image of a React app
Using Window Subsystem for Linux (WSL) via a Terminal window inside Visual Studio Code, to build the Docker image for the React app, the following docker command was used. You can verify whether this was successful by looking for the image within the visual interface of the Docker application or use the docker images terminal command.
docker image build -t example-app:latest .
How to create a container of your React app image
After running the Docker run command, the container should appear within the visual interface of the Docker application and you can access your React app via http://localhost:8000/
docker run -dp 8000:3000 --name example-container example-app:latest